Task Manager Fullstack
A task management app built with cutting-edge tech for fluid user experience 🚀
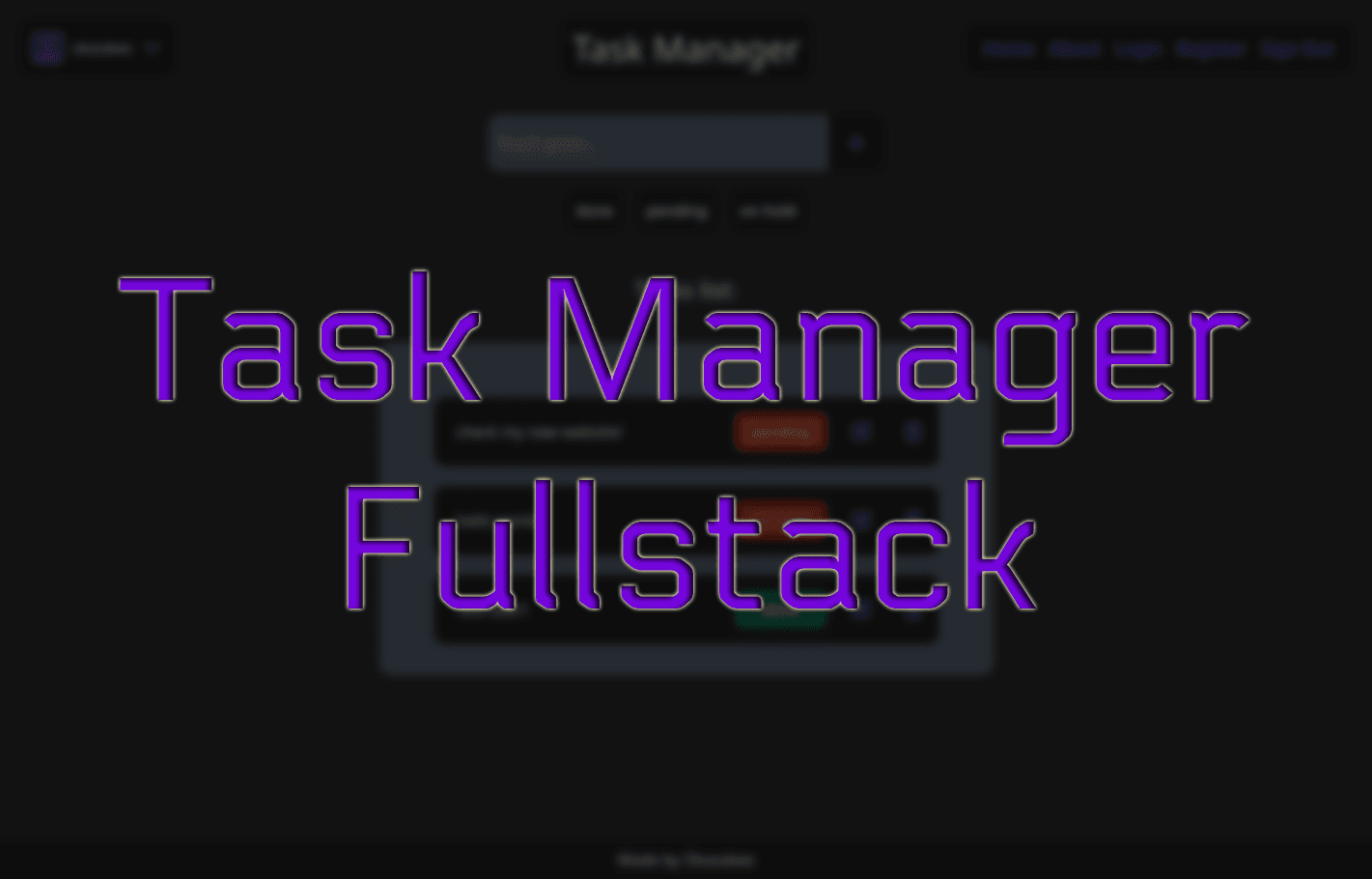
⚠️ Note that the Backend is hosted on render.com. This means that it might take some seconds before coming back online as per free tier limitation!
The Story
Every developer's journey includes building a task manager - it's almost a rite of passage. But instead of creating just another todo list, I saw an opportunity to explore the latest tech stack while solving a common problem. The goal? Create a task manager that's not only functional but also serves as a playground for modern web technologies and best practices.
What Makes It Special
Picture a task manager that's as responsive as your thoughts. Add a task? It's there instantly. Update its status? The UI updates seamlessly. Delete something? Gone without a page refresh. All this magic happens through the power of TanStack Query's real-time synchronization, making the app feel less like a web page and more like a native application. ✨
The Technical Craft
Core Features
- 🔄 Real-time Updates: Instant UI synchronization using TanStack Query
- 🎯 Clean Routing: Smooth navigation with TanStack Router
- 🎨 Modern UI: Sleek design with Tailwind CSS
- 🔧 Robust Backend: Express.js API with SQLite persistence
- 🚀 Lightning Fast: Built with Vite for optimal development experience
The Secret Sauce
Frontend Magic:
- Vite for blazing-fast development
- TanStack Query for efficient data fetching and caching
- TanStack Router for type-safe routing
- Tailwind CSS for responsive styling
- TypeScript for enhanced developer experience
Backend Power:
- Express.js for a robust API
- SQLite for reliable data storage
- Custom middleware for error handling
- RESTful architecture principles
Challenges & Solutions
The State Management Puzzle 🧩
- Challenge: Maintaining sync between server state and UI
- Solution: Implemented TanStack Query's powerful caching and invalidation system
- Result: Seamless real-time updates with optimistic UI changes
The TypeScript Architecture 🎯
- Challenge: Maintaining type safety across the full stack
- Solution: Created shared type definitions and robust interfaces
- Result: Enhanced developer experience with full-stack type safety
Technical Deep Dive
Frontend Architecture
Example of a task mutation using TanStack Query
Code
Copied!
const addTask = useMutation({
mutationFn: (newTask: Task) => {
return axios.post('/api/tasks', newTask)
},
onSuccess: () => {
queryClient.invalidateQueries({ queryKey: ['tasks'] })
}
})
Backend Structure
Task route handler with proper error handling
Code
Copied!
router.post('/tasks', async (req: Request, res: Response) => {
try {
const task = await db.create(req.body)
res.status(201).json(task)
} catch (error) {
handleError(error, res)
}
})
Behind the Scenes
This project was an exercise in balancing modern development practices with practical functionality. Every technical decision, from choosing Vite for the build system to implementing TanStack Query for state management, was made with both developer experience and end-user performance in mind.
What's Next?
- [ ] Task categories and tags
- [ ] Drag-and-drop task reordering